React is a JavaScript library created by Facebook for building user interfaces. React is used to build single page applications. It allows us to create reusable UI components.
It uses virtual DOM instead of Real DOM considering that Real DOM manipulations are expensive. React updates a virtual representation of the DOM in memory, then calculates the minimum number of changes necessary to update the actual DOM. This approach can be much faster than traditional DOM manipulation, which can involve many expensive operations.
React Hooks allows you to use State and other React features without writing a class. Hooks are the functions which “hook into” react state and and lifecycle features from functional components.
States is a built-in object that allows you to store and manage data within a component. Whenever a state changes, React will re-render the component to reflect the updated state
Props are basically properties and they are used to pass data between React components. React data flow between components is un-directional( from parent to child only) ,Props are used to communicate between the components
There are several built-in Hooks in React including useState, useEffect, and useRef:
Contents
useState
The useState Hook is one of the most commonly used Hooks in React. It allows you to track state in a functional components. It takes an initial state value as its argument and returns an array with two items – the current state value and the function to update it
Example 1:
import React, { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
function increaseCount(){
setCount(count+1);
}
function decreaseCount(){
setCount(count-1);
}
return (
<div>
<h1>The value of count is: {count}</h1>
<div>
<button onClick={increaseCount}>Increment</button>
<button onClick={decreaseCount}>Decrement</button>
</div>
</div>
)
}
export default Counter;
In this example, we’re using the useState Hook to create a count state variable with an initial value of 0. We’re then using the setCount function to increment and decrement the count variable whenever the button is clicked.
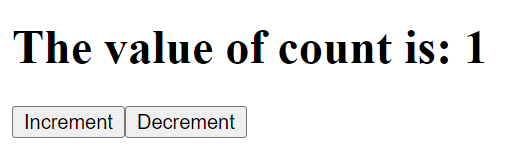
Example 2:
import React, { useState } from "react";
const Input = () => {
const [name, setName] = useState();
function handleChange(e){
setName(e.target.value);
}
return (
<div style={{display: 'flex', flexDirection: 'column', width: '35%'}}>
<h1>Name: {name}</h1>
<input type="text" onChange={handleChange}/>
</div>
)
}
export default Input;
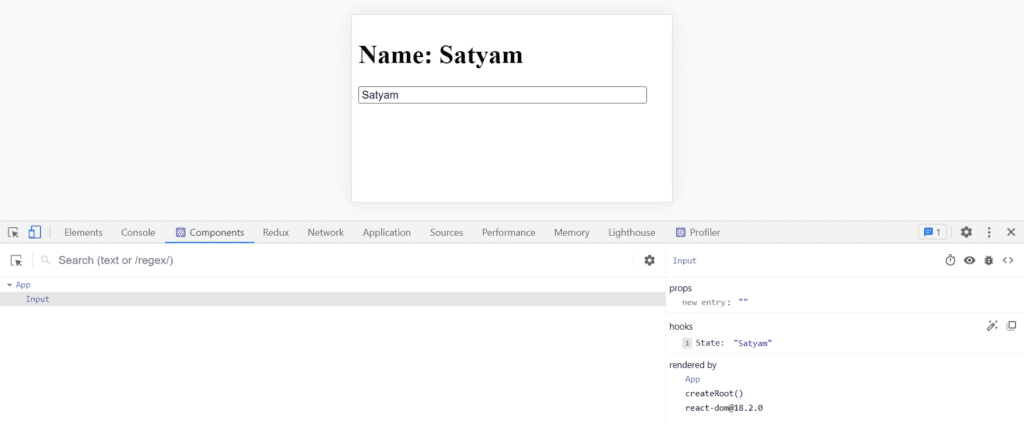
In this example, we’re using the useState Hook to create a state variable called ‘name’ with an initial value of an empty string. We’re then using the ‘setName’ function to update the ‘name’ variable whenever the value of the input field changes. Whenever the user types something in the input field, the ‘hand
Example 3:
import React from 'react'
import { useState } from 'react'
const Notes = () => {
const [notes, setNotes] = useState([]);
const [currNote, setCurrNote] = useState('');
function updateCurNote (e) {
setCurrNote(e.target.value);
}
return (
<>
<input type="text" onChange={updateCurNote} />
<button onClick={() => setNotes([...notes, currNote])}>Add Note</button>
<ul>
{notes.map((note, index) => (
<li key={index}>{note}</li>
))}
</ul>
</>
)
}
export default Notes;
useRef
The useRef hook is React hook that lets you reference a value that’s not needed for rendering. By using a ref, you can store the information between re-render and changing it doesn’t trigger a re-render(unlike state variables which triggers a re-render)
import React, { useRef } from "react";
const Input = () => {
const nameInputRef = useRef(null);
function handleSubmit(e){
e.preventDefault();
console.log(nameInputRef.current.value);
}
return (
<div style={{display: 'flex', flexDirection: 'column', width: '35%'}}>
<h1>Enter your name</h1>
<input type="text" ref={nameInputRef}/>
<button onClick={handleSubmit}>Submit</button>
</div>
)
}
export default Input;
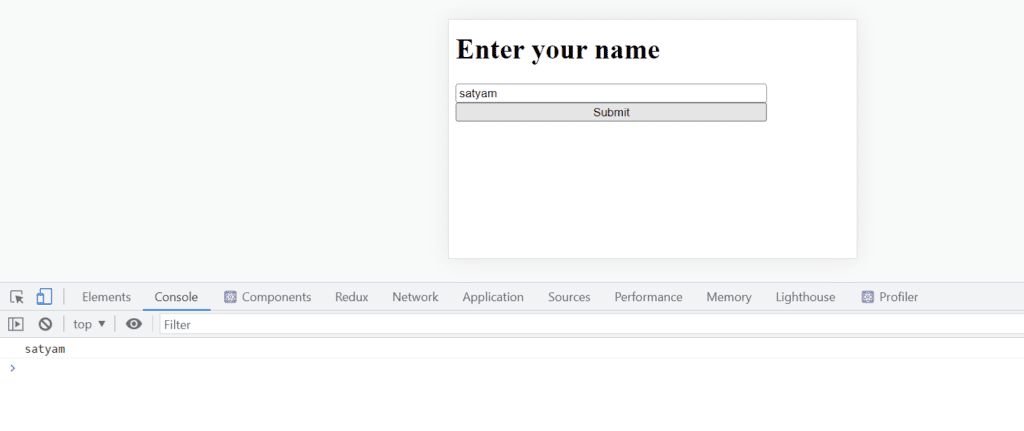
In this example, we’re using the useRef Hook to create a reference to a string value that is set to an empty string. We’re then using this reference to update and access the value of the input element without triggering a re-render of the component. When the submit button is click, we’re using the value of the nameInputRef.current.value to display message on console.
Hence, we can see that in useState, whenever there is a change in state, re-rendering occurs and in useRef, no re-rendering occurs on changing state but value of data retains.
useEffect
The useEffect Hook in React allows you to manage side effects in functional components. Side effects are actions that occur outside of the normal component rendering, such as fetching data from an API.
The useEffect Hook takes two arguments: a function that contains the side effect code, and an optional array of dependencies.
The function will be executed after the component has been rendered to the screen. The function can contain any code that you want to run as a side effect such as fetching data from an API or updating the DOM.
You can listen to various events by passing different values inside the second argument:
- pass nothing: Runs when the component mounts and on every re-renders.
- pass an empty array: Runs when the component mounts and not on re-renders.
- passing elements with state changes: Runs when the component mounts and the elements inside the array changes.
import React, { useEffect, useState } from "react";
const Input = () => {
const [counter, setCounter] = useState(0);
const [name, setName] = useState('');
function callThisOnUnmout() {
console.log('unmounting here');
}
useEffect(() => {
console.log(name, 'name updated');
return callThisOnUnmout;
}, [name]);
useEffect(() => {
console.log('first time mounted')
}, []);
useEffect(() => {
console.log(counter, 'counter updated');
}, [counter]);
useEffect(() => {
console.log('re-rendering happened');
});
return (
<div>
<p>{counter}</p>
<input type="text" onChange={(e) => setName(e.target.value)} />
<button onClick={() => setCounter(counter+1)}>Increment</button>
</div>
)
}
export default Input;
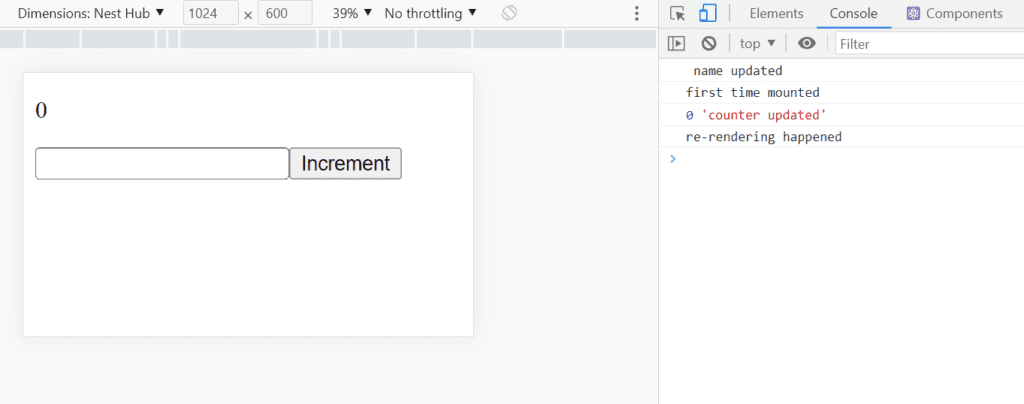
Now click on increment button
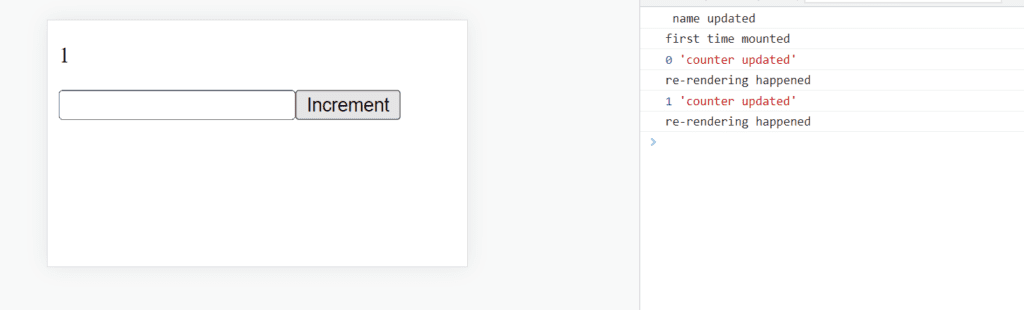
Now type something in input form
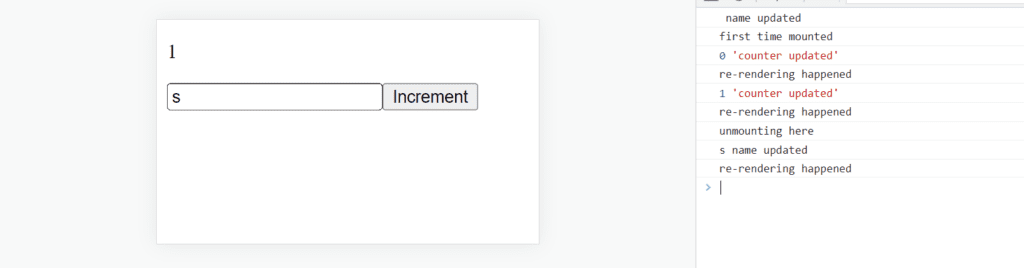
Let’s understand useState, useEffect, and useRef with the help of one minor project – News App
Create two files- NewsApp.js and News.js
NewsApp.js
import React, { useEffect, useRef, useState } from "react";
import News from "./News";
const NewsApp = () => {
const apiKey = "";
const [newsList, setNewsList] = useState([]);
const [query, setQuery] = useState('tesla');
const apiUrl = `https://newsapi.org/v2/everything?q=${query}&from=2023-03-09&sortBy=publishedAt&apiKey=${apiKey}`;
const queryInputRef = useRef(null);
useEffect(() => {
fetchData();
}, [query]);
async function fetchData() {
try{
const response = await fetch(apiUrl);
const jsonData = await response.json();
setNewsList(jsonData.articles);
}catch(e){
console.log(e,"error occured");
}
}
function handleSubmit(event){
event.preventDefault();
const queryValue = queryInputRef.current.value;
setQuery(queryValue);
}
return (
<div>
<h1 style={{fontSize: '2.4rem', textAlign: 'center'}}>Daily news</h1>
<form onSubmit={handleSubmit} style={{textAlign: 'center'}}>
<input type="text" ref={queryInputRef}/>
<input onClick={handleSubmit} type="submit" value="Search"/>
</form>
<div style={{display: "grid", gridTemplateColumns:"repeat(3,32%)", justifyContent: 'space-between', rowGap:"10px"}}>
{newsList.map((news) => {
return <News key={news.url} news={news} />
})}
</div>
</div>
)
}
export default NewsApp;
News.js
import React from 'react';
import './News.css';
const News = ({news}) => {
return (
<div className="news-card">
<img src={news.urlToImage} alt={news.title} />
<h2>{news.title}</h2>
<p>{news.description}</p>
<button className="btn-read-more" onClick={() => window.open(news.url)}>Read More</button>
</div>
)
}
export default News;
News.css
.news-card{
padding: 20px;
border: 2px solid #666;
}
img{
width: 100%;
margin-bottom: 5px;
}
h2{
margin-bottom: 10px;
}
p{
color: #333;
margin-bottom: 10px;
}
.btn-read-more{
width: 25%;
margin-inline: auto;
padding-block: 10px;
color: white;
background-color: black;
}
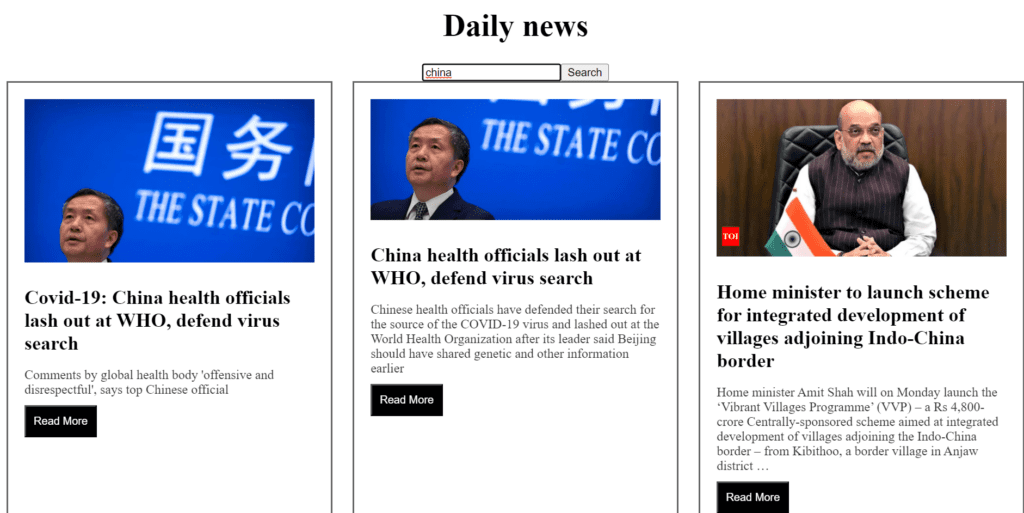
In conclusion, the useState, useEffect, and useRef Hooks are essential tools for managing state, side effects, and references in React functional components. The useState Hook allows you to create state variables that can be updated and synchronized with the UI. The useEffect Hook allows you to manage side effects such as data fetching and can clean up after the component is unmounted. The useRef Hook allows you to create a reference to a DOM element or any other value that persists across renders.
useSearchParams & useLocation
The `useLocation` hook in React Router is used to return the current location of a React component. The `useLocation` returns the current location as an object and comes with props such as
- pathname
- search
- key
- hash
- state
import { useLocation } from "react-router-dom";
const location = useLocation();
console.log(location);
For ex: http://localhost:3000/products/school/?name=bags#react
hash: '#react'
pathname: '/products/school/'
search: '?name=bags',
state: undefined
The useSearchParams
hook is used to read and modify the query string in the URL for the current location. Like React’s own useState
hook, useSearchParams
returns an array of two values: the current location’s search params and a function that may be used to update them.
https://example.com/search?q=react&lang=en
In this URL, q and lang are query parameters. The value of q is react and the value of lang is en. Query parameters are often used to filter or sort data in a web application.
import { useSearchParams } from 'react-router-dom';
const [searchParams, setSearchParams] = useSearchParams();
const q = searchParams.get('q');
const lang = searchParams.get('lang');
setSearchParams('q', 'react router');
setSearchParams({ q: 'react router', lang: 'fr' });
In short, useSearchParams is used to get and update search parameters (query parameters) while useLocation is used to get pathname and add search query after pathname.