Node.js is an open-source, cross-platform, server-side JavaScript runtime environment built on Chrome’s V8 JavaScript engine. It allows developers to use JavaScript to write server-side code. It provides an event-driven, non-blocking (asynchronous) I/O and cross-platform runtime environment for building highly scalable server-side applications using JavaScript.
Contents
Traditional Web Server Model
In the traditional web server model, a web server handles incoming HTTP requests from clients (such as web browsers) and responds with HTML, CSS, JavaScript, and other assets that make up a web page. When a client makes a request to a web server, the server creates a new thread or process to handle the request. This thread or process performs the necessary processing and generates the response, which is then sent back to the client.
One of the drawbacks of this model is that it can be resource-intensive, especially when handling a large number of concurrent connections. Each thread or process requires a certain amount of memory and processing power, and creating and managing a large number of threads or processes can put a strain on the server’s resources.
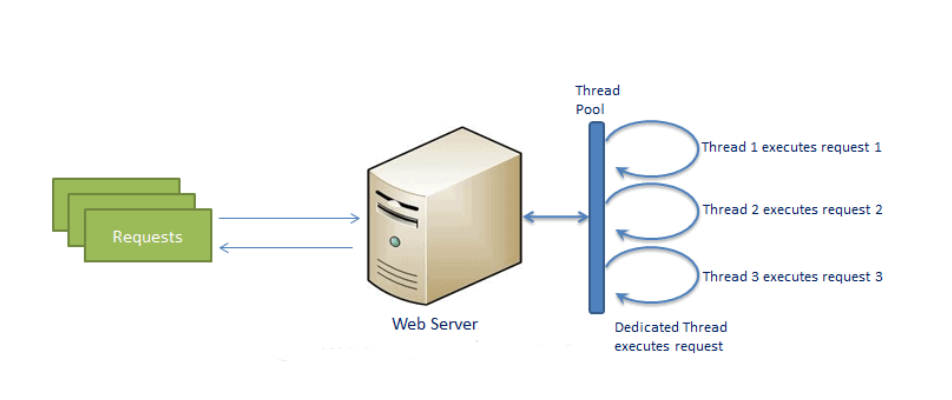
Node.js Process Model
Node.js runs in a single process and the application code runs in a single thread and thereby needs less resources than other platforms. All the user requests to your web application will be handled by a single thread and all the I/O work or long running job is performed asynchronously for a particular request. So, this single thread doesn’t have to wait for the request to complete and is free to handle the next request. When asynchronous I/O work completes then it processes the request further and sends the response.
The Node.js process model is based on an event-driven, non-blocking I/O architecture, which allows Node.js to handle a large number of concurrent connections with a single thread or process.
In Node.js, when a client makes a request to the server, the server creates an event loop that listens for incoming requests. When a request comes in, the event loop adds it to a queue, and then continues to listen for new requests.
The event loop processes the queue of incoming requests one by one, and each request is handled in a separate callback function. While waiting for I/O operations (such as reading from a file or querying a database) to complete, the event loop continues to process other requests in the queue. This means that while waiting for I/O operations to complete, the thread is not blocked and can handle other requests.
When an I/O operation is completed, a callback function is called, and the event loop adds the callback function to the queue of tasks to be processed. When the event loop gets to the callback function, it executes it, and then continues to process the rest of the queue.
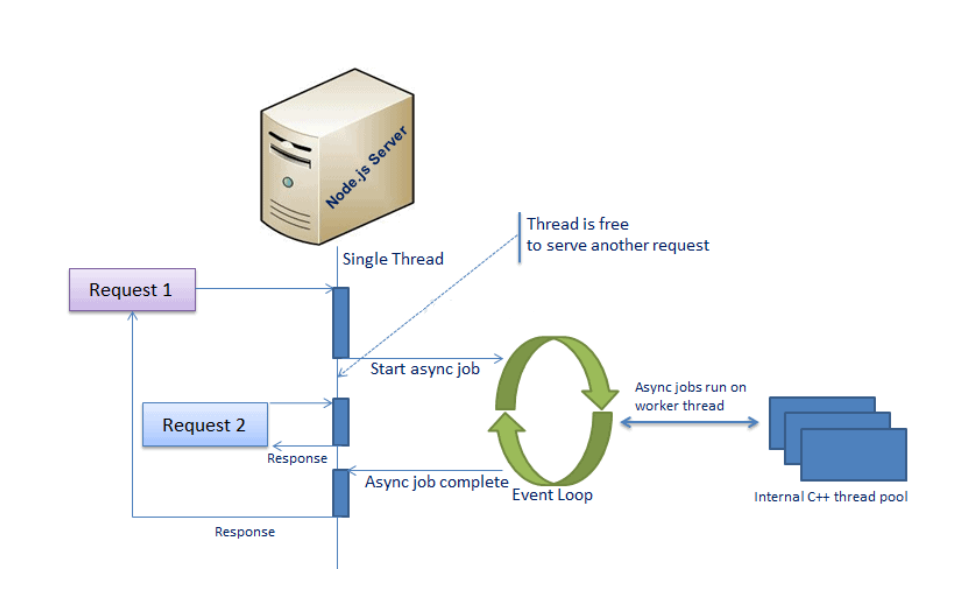
NPM
Node Package Manager (NPM) is a command line tool that installs, updates or uninstalls Node.js packages in your application. It is also an online repository for opensource Node.js packages. The node community around the world creates useful modules and publishes them as packages in this repository.
npm init -y
npm init -y is a command used to initialize a new npm project with default settings, without prompting the user for any input. The -y flag tells npm to automatically accept all default values for the project configuration.
npm install
npm install is a command used to install all the dependencies specified in a project’s package.json file.
npm update
npm update is a command used to update the packages in a project to their latest versions, as specified in the package.json file
npm install <package name>
npm install <package name> is a command used to install a specific package (along with its dependencies) in a Node.js project.
Example – How to create a Node.js HTTP server using the built-in http
module
var http = require("http");
http.createServer(function (request, response) {
response.writeHead(200, {'Content-Type': 'text/plain'});
response.end('Hello World');
}).listen(8081);
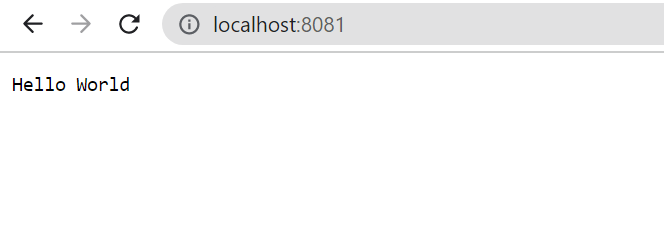
ExpressJS
Express.js is a web application framework for Node.js. It provides various features that make web application development fast and easy which otherwise takes more time using only Node.js.
Advantages of Express.js
- Makes Node.js web application development fast and easy.
- Easy to configure and customize.
- Allows you to define routes of your application based on HTTP methods and URLs.
- Includes various middleware modules which you can use to perform additional tasks
on request and response. - Allows you to define an error handling middleware.
- Allows you to create REST API server.
- Easy to connect with databases such as MongoDB, MySQL
To install express:
npm install -g express
const express = require('express');
const app = express();
app.get("/user", (req,res) => {
console.log("user was called");
res.send({
name: "Satyam",
age: 20,
})
})
app.listen(4000, () => {
console.log("listening on port 4000");
});
.env
The .env file is a configuration file used to store environment variables for an application.
Example:
DB_PASSWORD=123456789
API_KEY=123456789
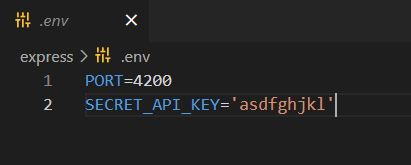
To use these environment variables in your Node.js application, you can load them into your process environment using a package dotenv
npm i dotenv
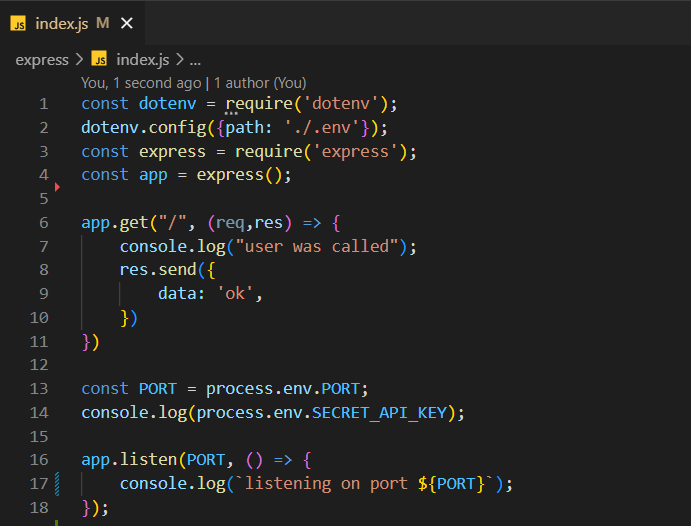
By loading environment variables from a .env file, you can keep sensitive information separate from your codebase and easily configure your application for different environments. It’s important to keep in mind that the .env file should not be committed to version control as it can contain sensitive information. Instead, you should add the .env file to your .gitignore file to ensure that it’s not accidentally committed to your repository.
API and HTTP Request Types
An API, which stands for Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate and interact with each other. APIs define how different software components should interact, making it easier for developers to use functionalities from one application in another without needing to understand the internal workings of the software.
HTTP Request Types:
- GET: The GET request is used to retrieve data from the server. It’s the most common type of request and is often used to fetch web pages, images, and other resources. When you enter a URL in your browser and hit Enter, your browser sends a GET request to the server to fetch the requested web page.
- POST: The POST request is used to send data to the server to be processed. It’s typically used to create new entities or edit already existing entities For example, when you fill out a login form and click “Submit,” your browser sends a POST request containing your login credentials to the server for verification.
- PUT: The PUT request is used to update or create a resource on the server.
- DELETE: The DELETE request is used to delete an entity from the server It’s used when you want to delete a file, a record, or any other resource from the server.
Express Router
In Express.js, a router is a middleware function that can be used to handle requests based on their URL paths. The router provides a way to organize your application’s routes into separate modules or files, making your code more modular and maintainable.
const express = require('express');
const router = express.Router();
router.get('/posts', (req, res) => {
// Code to display all blog posts
});
router.post('/posts', (req, res) => {
// Code to create a new blog post
});
router.get('/posts/:id', (req, res) => {
// Code to retrieve and display a specific blog post
});
router.put('/posts/:id', (req, res) => {
// Code to update a specific blog post
});
module.exports = router;
const express = require('express');
const blogRouter = require('./routes/blog');
const app = express();
app.use('/blog', blogRouter);
app.listen(3000, () => {
console.log('Server started on port 3000');
});
MVC Architecture
MVC (Model-View-Controller) is a design pattern commonly used in software engineering for building user interfaces. It divides the application into three interconnected components, each with its own responsibilities. The main goal of MVC is to split large applications into specific sections that have their own individual purpose.
- Model: Represents the data and the business logic of the application. It encapsulates the data and provides methods to interact with it, without worrying about how the data is displayed or how the user interacts with it.
- View: Represents the user interface of the application. It displays the data to the user and handles user input.
- Controller: Acts as an intermediary between the model and the view. It handles user input, updates the model accordingly, and then updates the view to reflect the changes made to the model.
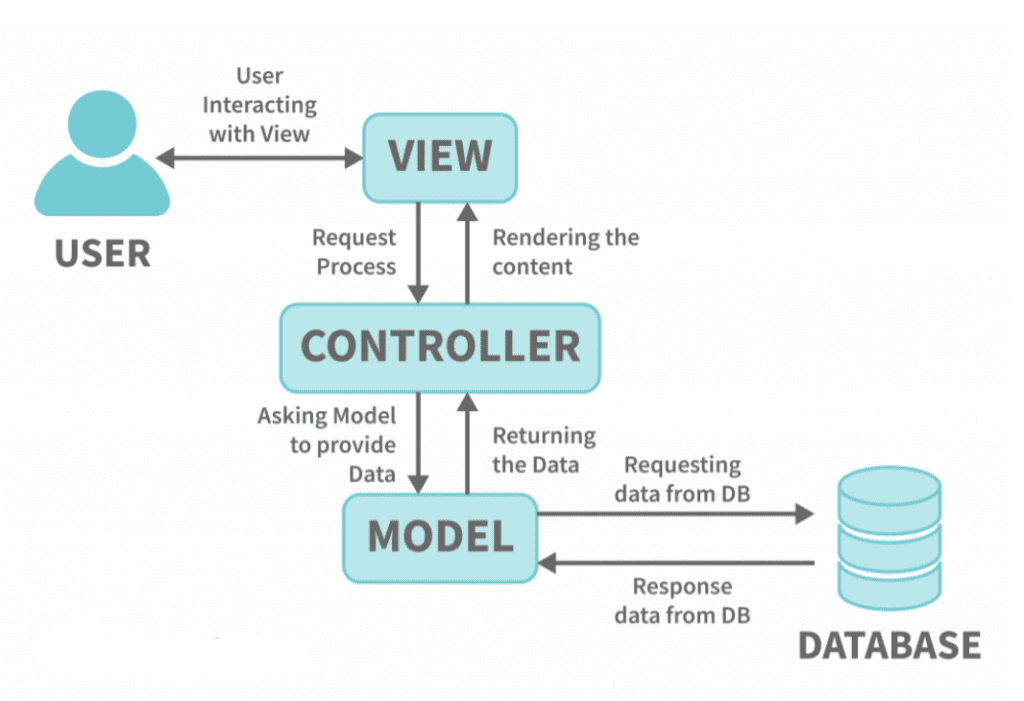
Here’s how the MVC pattern works in a web application:
- The user interacts with the View component, typically through a web browser or a mobile app.
- The View sends a request to the Controller, which handles the request and updates the Model if necessary.
- The Model updates its data and sends a notification to the Controller, which then updates the View with the new data.
- The updated View is then sent back to the user.
Middleware
Middleware is software that acts as a bridge between an application’s request and response objects. Middleware functions are executed in a pipeline, where each middleware function can manipulate the request and response objects before passing them on to the next middleware in the pipeline.
Some common middleware modules in Node.js include:
- Cookie-parser: A middleware module that parses cookies attached to the client request object.
- Morgan: A middleware module that logs HTTP requests to the console
- Express-session: A middleware module that enables session management in Express applications.
This example shows a middleware function with no mount path. The function is executed every time the app receives a request.
const express = require('express')
const app = express()
app.use((req, res, next) => {
console.log('Time:', Date.now())
next();
})
MongoDB
MongoDB is a rich open-source document-oriented and one of the widely recognised NoSQL database. It is written in C++ programming language
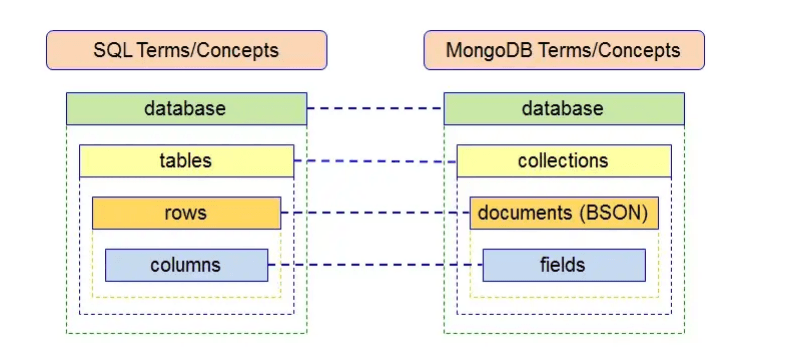
MongoDB supports many datatypes:
- String − This is the most commonly used datatype to store the data. String in MongoDB must be UTF-8 valid.
- Integer − This type is used to store a numerical value. Integer can be 32 bit or 64 bit depending upon your server.
- Boolean − This type is used to store a boolean (true/ false) value.
- Double − This type is used to store floating point values.
- Arrays − This type is used to store arrays or list or multiple values into one key.
- Timestamp − This can be handy for recording when a document has been modified or added.
- Object − This datatype is used for embedded documents.
- Null − This type is used to store a Null value.
- Date − This datatype is used to store the current date or time in UNIX time format. You can specify your own date time by
- creating object of Date and passing day, month, year into it.
- Object ID − This datatype is used to store the document’s ID.
- Binary data − This datatype is used to store binary data.
- Code − This datatype is used to store JavaScript code into the document.
- Regular expression − This datatype is used to store regular expression.
Mongoose
Mongoose is an object modeling tool for MongoDB and Node.js. What this means in practical terms is that you can define your data model in just one place, in your code. It allows defining schemas for our data to fit into, while also abstracting the access to MongoDB. This way we can ensure all saved documents share a structure and contain required properties.
npm i mongoose
Connect NodeJS with MongoDB Database using mongoose
const mongoose = require('mongoose');
const mongoUri = "mongodb+srv://satyam:QDietkOLnIf6T7pV@cluster0.xy2vuav.mongodb.net/?retryWrites=true&w=majority";
try{
mongoose.connect(mongoUrl, { useNewUrlParser: true, useUnifiedTopology: true }, () => {
console.log('mongodb connection established')
});
}catch(e){
console.log(e);
}
Note: Make sure to replace mongoUri with your mongoUri string
Blog on Getting started with MongoDB Atlas : Click here
Blog on Getting Started with MongoDB Community Server : Click here